CcodeTemplates
Alias_Compile/Run
Arguments_Read_Integer
Arguments_Read_Float
Arguments_Read_Options
Arguments_Printout
Convert_Aiff_File
Convert_BMP_File
Convert_GDSII_File
Final
File_Read_String/Data
File_IO
File_IO_double
File_IsAlpha_Or_Digit
File_Place_Get_Pointer
File_Place_Put_Pointer
File_Random_Access
File_Write_From_Keyboard
File_Write
File_Find_Replace
Function
Math_function.c
ReadLn_From_Keyboard
Read_In_Array
Simple
String_Append
Structures
==========Alias_Compile/Run========
donsauer$
alias r = "gcc test1.c
-o
test1
./test1 "
donsauer$
r
I'm a C
program
==========Simple========
================Test1.c=====================
#include
<stdio.h>
main
() { printf ("I'm a C program\n"); }
================Test1_compile=====================
donsauer$ cd
/Users/donsauer/Documents/KEY/KEY0/IDEA2IC/PlayWithXcode/CLanguageExamples
donsauer$ gcc test1.c
-o
test1
donsauer$ ./test1
I'm a C
program
==========Function========
================Test2.c=====================
#include <stdio.h>
int sum ( int x, int y );
main
()
{ int theSum = sum (10, 11);
printf ( "Sum: %i\n", theSum );
}
int sum ( int x, int y )
{ return x + y;
}
================Test2_compile=====================
donsauer$ gcc test2.c
-o
test2
donsauer$ ./test2
Sum: 21
==========Math_function.c========
================math_functions.h=====================
int sum
(int x, int y);
float average
(float x, float y, float z);
================math_functions.c=====================
int sum (int x, int
y)
{ return (x + y);
}
float average
(float x, float y, float z)
{ return (x + y + z) / 3;
}
================test3.c===============================
#include <stdio.h>
#include "math_functions.h"
main ()
{ int theSum = sum
(8, 12);
float theAverage = average
(16.9, 7.86, 3.4);
printf ("the sum is: %i ", theSum);
printf ("and the average is: %f \n", theAverage);
printf ("average casted to an int is: %i \n", (int)theAverage);
}
================Test3_compile=====================
donsauer$ gcc test3.c
math_functions.c -o
test3
donsauer$ ./test3
the sum is:
20 and the average is: 9.386666
average
casted to an int is: 9
==========Structures===========
================song.h=====================
typedef struct
{ int lengthInSeconds;
int yearRecorded;
} Song;
Song
make_song
(int seconds, int year);
void display_song (Song
theSong);
================song.c=====================
#include <stdio.h>
#include "song.h"
Song
make_song
(int
seconds, int year)
{ Song newSong;
newSong.lengthInSeconds = seconds;
newSong.yearRecorded = year;
display_song
(newSong);
return newSong;
}
void display_song (Song theSong)
{ printf ("the song is %i seconds long ", theSong.lengthInSeconds);
printf ("and was made in %i\n", theSong.yearRecorded);
}
================test4.c=====================
include <stdio.h>
#include "song.h"
main ()
{ Song firstSong = make_song
(210, 2004);
Song secondSong = make_song
(256, 1992);
Song thirdSong = { 223, 1997 };
display_song
( thirdSong );
Song fourthSong = { 199, 2003
};
}
================Test4_compile=====================
donsauer$ gcc
test4.c song.c -o test4
donsauer$ ./test4
the song is
210 seconds long and was made in 2004
the song is
256 seconds long and was made in 1992
the song is
223 seconds long and was made in 1997
==========Final=================
================final.c=====================
#include <stdio.h>
#include "math_functions.h"
#include "song.h"
main ()
{ const int numberOfSongs = 3;
printf ("total number of songs will be: %i\n", numberOfSongs);
int i;
for (i = 0; i < numberOfSongs; i++)
{ printf ("loop trip %i ", i);
} printf ("\n");
Song song1 = make_song (223, 1998);
Song song2 = make_song (303, 2004);
Song song3 = { 315, 1992 };
display_song
(song3);
int combinedLength = sum
(song1.lengthInSeconds, song2.lengthInSeconds);
printf ("combined length of song1 and song2 is %i\n",
combinedLength);
float x = (float) song1.lengthInSeconds;
float y = (float) song2.lengthInSeconds;
float z = (float) song3.lengthInSeconds;
float averageLength;
averageLength = average
(x, y, z);
printf ("average length is: %f as a float ", averageLength);
printf ("and %i as an int\n", (int)
averageLength);
}
================Final_compile=====================
donsauer$ gcc
final.c song.c math_functions.c
-o final
donsauer$ ./final
total number of songs will be: 3
loop trip 0 loop trip 1 loop
trip 2
the song is 223 seconds long
and was made in 1998
the song is 303 seconds long
and was made in 2004
the song is 315 seconds long
and was made in 1992
combined length of song1 and
song2 is 526
average length is: 280.333344
as a float and 280 as an int
=========File_Read_String/Data==========
================test3.cpp=====================
#include <iostream>
#include <fstream>
using namespace std;
int main()
{ ifstream
in("hello.aiff",
ios::in | ios::binary);
if(!in)
{ cout << "Cannot open input
file.\n";
return 1;
} int num;
char str[80];
in.read(str, 4);
str[4] =
'\0';
// null terminate str
cout << str <<
"\n "
;
// print double and string
in.read((char *) &num,
sizeof(int));
// reads from file into
int as LEnd..not BEnd
cout << num <<
"\n "
;
// print double and string
in.close();
return 0;
}
================test3_compile=====================
donsauer$ g++
-v -Wall test3.cpp
-o test3
donsauer$ ./test3
FORM
788007680
00000_04_bEnd_charac____IFF-Header
= FORM {464F524D}
00004_04_bEnd_unsign____File-Size
= 784430 {000BF82E}
788007680
{000BF82E}=>{2EF80B00}
============File_IO==========
================test2.c=====================
#include <fstream>
#include <iostream>
using namespace std;
int main()
{ std::ofstream ofile;
ofile.open("ex.txt", ios::out);
//opens file as output
int
x=1;
ofile<<x;
ofile.close();
//ex.txt is now "1"
std::ifstream ifile;
ifile.open("ex.txt",ios::in);
//opens file as input
int a;
ifile>>a;
//a now equals 1
a=2;
ifile.close();
ofile.open("ex.txt", ios::out|ios::trunc);
//opens file as output ex.txt empty due to
ios::trunc
ofile<<x;
//write 1 to file..ex.txt is now "1"
ofile.close();
ofile.open("ex.txt", ios::out|ios::app);
//open file as output and appends
ofile<<a;
//write 2 to file ..ex.txt is now "12"
ofile.close();
ofile.open("ex.txt", ios::in|ios::out|ios::ate); //open file as output
ofile<<x;
//write 1 to file..ex.txt is now "121"
ofile.close();
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2
============File_IO_double==========
================test2.c=====================
#include <iostream>
#include <fstream>
using namespace std;
int main()
{ ofstream out("test", ios::out | ios::binary);
if(!out)
{ cout << "Cannot open output file.\n";
return 1;
} double nums[4] = {1.1, 2.2, 3.3, 4.4
};
out.write((char *) nums, sizeof(nums));
//writes as Lend
out.close();
ifstream in("test", ios::in | ios::binary);
if(!in)
{ cout << "Cannot open input file.\n";
return 1;
} in.read((char *) &nums,
sizeof(nums));
int i;
for(i = 0; i <4; i++) cout << nums[ i ] << ' ';
// 1.1 2.2 3.3 4.4
cout << '\n';
cout << in.gcount()
<< " characters read\n"; // 32 characters = bytes read
in.close();
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2
1.1
2.2 3.3 4.4
32 characters read
===========File_IsAlpha_Or_Digit==========
================test2.c=====================
#include <iostream>
#include <fstream>
#include <cctype>
using namespace std;
int main()
{ char ch;
ofstream out("test", ios::out | ios::binary);
if(!out)
{ cout << "Cannot open output file.\n";
return 1;
} char str[80], *p;
out << 123 << "this is a
test" << 23;
out << "Hello there!" << 99
<< "sdf" << endl;
out.close();
ifstream in("test", ios::in | ios::binary);
if(!in)
{ cout << "Cannot open input file.\n";
return 1;
} do
{ p = str;
ch = in.peek();
if(isdigit(ch)) {
while(isdigit(*p=in.get()))
p++;
in.putback(*p);
*p =
'\0';
// null terminate the string
cout << "Integer: " << atoi(str);
} else if(isalpha(ch))
// read a string
{ while(isalpha(*p=in.get())) p++;
in.putback(*p);
// return char to stream
*p =
'\0';
// null terminate the string
cout << "String: " << str;
} else
in.get();
// ignore
cout << '\n';
} while(!in.eof());
in.close();
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2
Integer: 123
String: this
String: is
String: a
String: test
Integer: 23
String: Hello
String: there
Integer: 99
String: sdf
================test=====================
123this is a test23Hello there!99sdf
===========File_Place_Get_Pointer==========
================test2.c=====================
##include <iostream>
#include <fstream>
#include <cstdlib>
using namespace std;
int main(int argc, char *argv[])
{ char ch;
if(argc!=3)
{ cout << "Usage: LOCATE <filename> <loc>\n";
return 1;
} ifstream in(argv[1], ios::in | ios::binary);
if(!in)
{ cout << "Cannot open input file.\n";
return 1;
} in.seekg(atoi(argv[2]),
ios::beg);
//places “get” pointer at argv[2] relative from beginning
while(!in.eof())
{ in.get(ch);
cout << ch;
} in.close();
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2 test
his is a test23Hello there!99sdf
================test=====================
123this is a test23Hello there!99sdf
===========File_Place_Put_Pointer==========
================test2.c=====================
#include <iostream>
#include <fstream>
#include <cstdlib>
using namespace std;
int main(int argc, char *argv[])
{ if( argc != 4)
{
cout << "Usage: CHANGE <filename> <character> <char>\n";
// ./test2 test t d
return 1;
} fstream out(argv[1], ios::in | ios::out | ios::binary);
if(!out)
{ cout << "Cannot open file.";
return 1;
} out.seekp(atoi(argv[ 2 ]), ios::beg);
//positions at val of argv[2] relative to begininng
out.put(*argv[ 3 ]);
out.close();
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2 test 5 d
================test=====================
123thds is a test23Hello there!99sdf
===========File_Random_Access==========
================test2.c=====================
#include <iostream>
#include <fstream>
#include <cstdlib>
using namespace std;
int main(int argc, char *argv[])
{ if(argc != 3)
{ cout << "Usage: CHANGE <filename> <byte>\n";
// ./test2 test 7
return 1;
} fstream out(argv[1], ios::in | ios::out | ios::binary);
if(!out) {
cout << "Cannot open file.\n";
return 1;
} out.seekp(atoi(argv[2]), ios::beg);
//sets put pointer argv[2] relative to beginning:
out.put('X');
out.close();
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2 test 7
================test=====================
123thdsXis a test23Hello there!99sdf
===========File_Write_From_Keyboard==========
================test2.c=====================
#include <iostream>
#include <fstream>
using namespace std;
int main(int argc, char *argv[])
{ char ch;
if(argc!=2)
{ cout << "Usage: WRITE <filename>\n";
return 1;
} ofstream out(argv[1], ios::out | ios::binary);
if(!out)
{ cout << "Cannot open file.\n";
return 1;
} cout << "Enter a $ to stop\n";
do
{ cout << ": ";
cin.get(ch);
// gets ch from cin
(keyboard)
out.put(ch);
// puts ch into
out
} while
(ch!='$');
out.close();
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2
tt.txt
Enter a $ to stop
: This is a Test $
: : : : : : : : : : : : : : :
================tt=====================
This
is a Test $
===========File_Write==========
================test2.c=====================
#include <fstream>
using namespace std;
int main ()
{ long pos;
ofstream outfile;
outfile.open ("test.txt");
outfile.write ("This is an apple",16); // This
is an apple , 16 char
pos=outfile.tellp();
// get position of put pointer
outfile.seekp
(pos-7);
// set position of put pointer
outfile.write ("
sam",4);
// This is a sample
outfile.close();
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2
================test.txt=====================
This
is a sample
================write2file.c=====================
#include <fstream>
#include <iostream>
using namespace std;
int main()
{ std::ofstream ofile;
ofile.open("ex.txt", ios::out); //opens file as output
int
x=1;
ofile<<x;
ofile.close();
//ex.txt is now "1"
}
/*
donsauer$ g++ -v write2file.c -o write2file
donsauer$ ./write2file
*/
===========File_Find_Replace==========
================test2.c=====================
#include <iostream>
#include <fstream>
using namespace std;
int main(int argc, char *argv[])
{ if(argc!=3)
{ cout << "Usage: CONVERT <input> <output>\n";
return 1;
} ifstream fin(argv[1]); //
open input file
ofstream fout(argv[2]); // create output file
if(!fout)
{ cout << "Cannot open output file.\n";
return 1;
} if(!fin)
{ cout << "Cannot open input file.\n";
return 1;
} char ch;
fin.unsetf(ios::skipws);
// do not skip spaces
while(!fin.eof())
{ fin >> ch;
if(ch==' ') ch = '|';
if(!fin.eof()) fout << ch;
} fin.close();
fout.close();
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2
test.txt test2.txt
================test2.txt=====================
This|is|a|Test|$
===========ReadLn_From_Keyboard==========
================test2.c=====================
#include <iostream>
#include <fstream>
using namespace std;
int main()
{ char str[80];
cout << "Enter your name: ";
cin.getline(str, 79);
// reads one line at a time
cout << str << '\n';
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2
Enter your name: don sauer
don sauer
================test2_alternative=====================
#include
<iostream>
using namespace std;
int main()
{
int number;
cout << "Please enter an integer: ";
cin >> number;
cout << uppercase
<< " octal \t
decimal \t hexadecimal\n "
<< oct << number
<< " \t "
<< dec << number
<< " \t "
<< hex << number
<< endl;
return 0;
}
/*
donsauer$ g++ -v test2.c -o test2
donsauer$ ./test2
alias r ='g++ -v test2.c -o test2
./test2'
don-sauers-macbook-pro:~ donsauer$ ./test2
Please enter an integer: 34
octal decimal
hexadecimal
42
34 22
*/
===========Arguments_Read_Integer==========
================test2.c=====================
#include <stdio.h>
#include <string.h>
main(int argc, char *argv[])
{ int inp;
if (argc !=2 )
{ printf("usage: a.out number \n"); // ./test2 1
//exist(0);
}
inp = atoi(argv[1]);
printf("%s\n",argv[1]); // 1
printf("%d\n",inp); // 1
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2 1
1
1
===========Arguments_Read_Float==========
================test2.c=====================
include <stdio.h>
#include <string.h>
main(int argc, char *argv[])
{ float inp;
int inp2;
if (argc !=2 )
{ printf("usage: a.out number \n"); //
./test2 1.0
//exist(0);
}
inp = atof(argv[1]);
inp2 = atoi(argv[1]);
printf("%s\n",argv[1]); // 1.0
printf("%f\n",inp); //
-1073744384.000000
printf("%d\n",inp2); // 1
inp = (float) inp2;
printf("%f\n",inp); //
1.000000
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2
1.0
1.0
-1073744384.000000
1
1.000000
===========Arguments_Read_Options==========
================test2.c=====================
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[])
{ int
i;
// ./test2 -a -b -d name
for (i = 1; i < argc; i++)
{ if (argv[i][0] ==
'-')
//Look for -a or -d in command line
if (argv[i][1] ==
'a')
puts
("Found -a"); // Found -a
else if (argv[i][1] ==
'd') puts ("Found -d"); // Found -d
else printf
(
"Unknown switch %s\n", argv[i]); // Unknown switch -b
else if (strcmp(argv[i], "name") ==
0) puts ("Found name"); // Found name
}
return(0);
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer ./test2
-a -b -d name
Found -a
Unknown switch -b
Found -d
Found name
===========Arguments_Printout==========
================test2.c=====================
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char **argv)
{ while(argc--)
printf("%s\n", *argv++);
exit(EXIT_SUCCESS);
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer$ ./test2
abcde text hello
./test2
abcde
text
hello
% prog 6 -why
file.c
//then the value of argc is 4 (four)
argv
---
--- -------------------
| -|---->
| -|----> | p | r | o | g |\0
|
//argv[0] is pointer to string "prog". the cc prog
---
|---| -------------------
| -|----> | 6 |\0
|
//argv[1] is the (pointer to the) string "6".
|---| -------------------
| -|----> | - | w | h | y |\0
|
//argv[2] is the (pointer to the) string "-why".
|---| ---------------------------
| -|----> | f | i | l | e | . | c |\0 |
//argv[3] is the (pointer to the) string "file.c".
|---| ---------------------------
character '\0' is a standard
way (in C code) to denote the NULL character.
===========String_Append==========
================test2.c=====================
#include <iostream>
using std::cout;
using std::endl;
#include <string>
using std::string;
int main()
{ string string1( "cat" );
string string3;
string3.assign( string1
);
//string1: cat
cout << "string1: " << string1
<< "\nstring3: "
<< string3 << "\n\n"; //string3: cat
string3 += "pet";
string3.append( string1, 1, string1.length() - 1 );
cout << "string1: " << string1
<< "\nstring3: "
<< string3 << "\n\n"; //string1: cat string3: catpetat
return 0;
}
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer ./test2
string1: cat
string3: cat
string1: cat string3: catpetat
===========Read_In_Array========
================test2.c=====================
int main() {
int
a[30][10];
// declares an int array of 30 rows and 10 columns.
char ticTacToe[3][3]; // declares three rows and three columns of chars.
char ticTacToeBoard[3][3] = {{'x', 'x', 'o'},
{'o', 'o', 'x'},
{'x', 'o', ' '}
};
int i,j;
for (int j=0; j<3; j++)
{ for (int i=0; i<3; i++)
{ cout << ticTacToeBoard[j][i] << " ";
}
cout << " \n";
}
return 0;
}//end main
================test2_compile=====================
donsauer$ g++ -v
test2.c -o test2
donsauer ./test2
x x
o
o
o x
x o
============Convert_AIFF_2_Text========
============aiff2txt.c=====================
#include
<iostream>
#include
<fstream>
#include
<math.h>
using namespace std;
ifstream::pos_type getPointer;
const
char
*fname;
char
str[60];
int
Int_Sign4( int first, int second, int third, int forth ) ;
int
Int_USign4( int first, int second, int third, int forth );
int
Int_USign2( int first, int second );
int
Int_Sign2( int first, int second );
double
Extend_10(int X0,int X1,int X2,int X3,int X4,int X5,int X6,int X7,int
X8,int X9 );
int
main (int argc, char * const argv[])
{
int
val , i , filesize ,numbframes, NumbChan ;
double
extendVal, sampleRate, time ;
std::ofstream ofile;
if
(argc !=2 )
{
printf(
"usage ./aiff2txt
file2convert.aiff \n");
exit(0);
}
ofile.open( "converted_aiff.txt",ios::out);
int x=33;
fname
=
argv[1];
cout
<<" "<< fname << " " ;
ofile
<<"filename = "<< fname <<
" \n" ;
ifstream
file (fname, ios::in|ios::binary|ios::ate);
if
(file.is_open())
{ getPointer =
file.tellg();
cout
<< " file size is " <<
getPointer << " \n";
ofile
<< "file size = " << getPointer << " \n";
filesize
=
getPointer;
file.seekg
( 0,
ios::beg);
// repositions
get pointer to beginning
}
cout
<< "ADDR
TYPE
VALUE \n";
ofile
<< "ADDR
TYPE
VALUE \n";
getPointer
=
file.tellg();
cout
<< getPointer << "
IFF_HEADER
";
ofile
<< getPointer << "
IFF_HEADER ";
file.read(str,4); str[4]=
'\0'; //
strings end with \0
cout
<<" " << str << " \n" ;
ofile
<<" " << str << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << "
FILE_SIZE
";
ofile
<< getPointer << "
FILE_SIZE ";
file.read(str,4);
val
=
Int_USign4( str[0], str[1], str[2], str[3] );
cout
<<" " << val << " \n" ;
ofile
<<" " << val << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << "
TYPE_AIFF
";
ofile
<< getPointer << "
TYPE_AIFF ";
file.read(str,4); str[4]=
'\0';
cout
<<" " << str << " \n" ;
ofile
<<" " << str << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << " COMMON_CHUNK";
ofile
<< getPointer << "
COMMON_CHUNK";
file.read(str,4); str[4]=
'\0';
cout
<<" " << str << " \n" ;
ofile
<<" " << str << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << " CHUNK_SIZE
";
ofile
<< getPointer << " CHUNK_SIZE
";
file.read(str,4);
val
=
Int_USign4( str[0], str[1], str[2], str[3] );
cout
<<" " << val << " \n" ;
ofile
<<" " << val << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << "
NUMB_CHAN
";
ofile
<< getPointer << "
NUMB_CHAN ";
file.read(str,2);
val
=
Int_USign2( str[0], str[1] );
cout
<<" " << val << " \n" ;
ofile
<<" " << val << " \n" ;
NumbChan
= val;
getPointer
=
file.tellg();
cout
<< getPointer << "
NUMB_FRAM
";
ofile
<< getPointer << "
NUMB_FRAM ";
file.read(str,4);
val
=
Int_USign4( str[0], str[1], str[2], str[3]);
numbframes
= val;
cout
<<" " << val << " \n" ;
ofile
<<" " << val << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << "
SAMP_SIZE
";
ofile
<< getPointer << "
SAMP_SIZE ";
file.read(str,2);
val
=
Int_USign2( str[0], str[1] );
cout
<<" " << val << " \n" ;
ofile
<<" " << val << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << "
SAMP_RATE
";
ofile
<< getPointer << "
SAMP_RATE ";
file.read(str,10);
extendVal
=
Extend_10(str[0],str[1],str[2],str[3],str[4],str[5],str[6],str[7],str[8],str[9]
);
cout
<<" " << extendVal << " \n" ;
ofile
<<" " << extendVal << " \n" ;
sampleRate
= extendVal;
getPointer
=
file.tellg();
cout
<< getPointer << " SSND_CHUNK
";
ofile
<< getPointer << " SSND_CHUNK
";
file.read(str,4); str[4]=
'\0';
cout
<<" " << str << " \n" ;
ofile
<<" " << str << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << " CHUNK_SIZE
";
ofile
<< getPointer << " CHUNK_SIZE
";
file.read(str,4);
val
=
Int_USign4( str[0], str[1], str[2], str[3]);
cout
<<" " << val << " \n" ;
ofile
<<" " << val << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << "
OFFSET
";
ofile
<< getPointer << "
OFFSET ";
file.read(str,4);
val
=
Int_USign4( str[0], str[1], str[2], str[3]);
cout
<<" " << val << " \n" ;
ofile
<<" " << val << " \n" ;
getPointer
=
file.tellg();
cout
<< getPointer << " BLOCK_SIZE
";
ofile
<< getPointer << " BLOCK_SIZE
";
file.read(str,4);
val
=
Int_USign4( str[0], str[1], str[2], str[3]);
cout
<<" " << val << " \n" ;
ofile
<<" " << val << " \n" ;
for
(i=0; i< numbframes -4 ; i++)
{ getPointer =
file.tellg();
file.read(str,2);
val
=
Int_Sign2( str[0], str[1] );
time
=
(double) i/ sampleRate;
ofile
<< time <<" " << val ;
if
( NumbChan > 1 )
{ file.read(str,2);
val
=
Int_Sign2( str[0], str[1] );
ofile
<<" " << val ;
}
ofile
<< " \n" ;
}
ofile.close();
cout
<<"Output is stored in
<converted_aiff.txt> \n" ;
return 0;
}
double
Extend_10(int
X0,int X1,int X2,int X3,int X4,int X5,int X6,int X7,int
X8,int X9 )
{
if
( X0 <0 ) X0 = X0+256;
if
( X1 <0 ) X1 = X1+256;
if
( X2 <0 ) X2 = X2+256;
if
( X3 <0 ) X3 = X3+256;
if
( X4 <0 ) X4 = X4+256;
if
( X5 <0 ) X5 = X5+256;
if
( X6 <0 ) X6 = X6+256;
if
( X7 <0 ) X7 = X7+256;
if
( X8 <0 ) X8 = X8+256;
if
( X9 <0 ) X9 = X9+256;
int
exp , i ,bit,testbit, scalebit;
exp
= (X0 & 127); //
mask = 01111111
exp
=
0;
//cout << " exp "<< exp <<"
<< \n" ;
for
(i=1; i< 8 ; i++)
{ bit
=
(int) pow(2,15-i);
exp
=
exp + (X0 & bit);
}
for
(i=0; i< 8 ; i++)
{ bit
=
(int) pow(2,7-i);
exp
=
exp + (X1 & bit);
}
exp
= exp +1; // add offset
double
matval,matvalb , value;
matval
= 0;
for
(i=0; i< 8 ; i++)
{ bit
=
pow(2,7-i);
scalebit =
pow(2,i+3);
testbit
= (X2 &
bit);
matvalb
= (double)
8*(X2 & bit)/(bit*scalebit);
matval
= matval +
matvalb;
matvalb
= (double)
8*(X3 & bit)/(256*bit*scalebit);
matval
= matval +
matvalb;
matvalb
= (double)
8*(X4 & bit)/(256*256*bit*scalebit);
matval
= matval +
matvalb;
} value
=
matval*pow(2,exp);
return value;
}
int
Int_Sign2(
int first, int second )
{
int
numb ;
if
( second <0 ) second = second+256;
numb
=
256*first + second;
return numb;
}
int
Int_USign2(
int first, int second )
{
int
numb ;
if
( first <0 ) first = first+256;
if
( second <0 ) second = second+256;
numb
=
256*first + second;
return numb;
}
int
Int_USign4(
int first, int second, int third, int forth )
{
int
numb ;
if
( first <0 ) first = first+256;
if
( second <0 ) second = second+256;
if
( third <0 ) third = third +256;
if
( forth <0 ) forth = forth +256;
numb
=
256*(256*(256*first + second)+third )+forth;
return numb;
}
int
Int_Sign4(
int first, int second, int third, int forth )
{
int
numb ;
if
( second <0 ) second = second+256;
if
( third <0 ) third = third +256;
if
( forth <0 ) forth = forth +256;
numb
=
256*(256*(256*first + second)+third )+forth;
// cout << " " << first << " " <<
second<< " " << third<< " " << forth << "
\n";
return numb;
}
==========aiff2txt.c_compile/RUN================
donsauer$ g++ -v aiff2txt.c -o aiff2txt
donsauer$ ./aiff2txt 0.1uf.aiff
0.1uf.aiff file size
is 910
ADDR TYPE
VALUE
0 IFF_HEADER FORM
4 FILE_SIZE 902
8 TYPE_AIFF AIFF
12 COMMON_CHUNK COMM
16 CHUNK_SIZE 18
20 NUMB_CHAN 2
22 NUMB_FRAM 214
26 SAMP_SIZE 16
28 SAMP_RATE 96000
38 SSND_CHUNK SSND
42 CHUNK_SIZE 864
46 OFFSET 0
50 BLOCK_SIZE 0
Output is stored in <converted_aiff.txt>
===========converted_aiff.txt===================
filename = 0.1uf.aiff
file size = 910
ADDR TYPE
VALUE
0 IFF_HEADER FORM
4 FILE_SIZE 902
8 TYPE_AIFF AIFF
12 COMMON_CHUNK COMM
16 CHUNK_SIZE 18
20 NUMB_CHAN 2
22 NUMB_FRAM 214
26 SAMP_SIZE 16
28 SAMP_RATE 96000
38 SSND_CHUNK SSND
42 CHUNK_SIZE 864
46 OFFSET 0
50 BLOCK_SIZE 0
0 -7348 372
1.04167e-05 -7263 303
2.08333e-05 -7196 247
3.125e-05 -7218 187
4.16667e-05 -7271 117
5.20833e-05 -7274 41
6.25e-05 -7230 -32
7.29167e-05 -7189 -98
8.33333e-05 -7194 -163
9.375e-05 -7215 -229
0.000104167 -7224 -296
0.000114583 -7201 -357
0.000125 -7174 -415
0.000135417 -7169 -475
0.000145833 -7187 -544
0.00015625 -7205 -616
0.000166667 -7193 -683
0.000177083 -7160 -736
0.0001875 -7149 -777
0.000197917 -7171 -819
etc....
============Convert_GDSII============
==================GDS2_BIN2TXT_main.cpp=============================
#include
<iostream>
#include
<fstream>
#include
<math.h>
using namespace std;
ifstream::pos_type getPointer;
char
* memblock;
unsigned
Int_2u(
unsigned hi, unsigned lo );
int
Numb_Bytes(int
hi, int lo );
int
Int_Sign4(
int first, int second, int third, int forth ) ;
double
Real_8(
int X0, int X1, int X2, int X3,int X4, int
X5, int X6, int X7 );
string
CodeID(
int hi, int lo );
char
str2[60];
const
char
*fname;
int
main (int argc, char * const argv[])
{ fname
=
"TEST.SF";
//Rename
files nmae.
cout
<<""<< fname << " is the file being read \n" ;
ifstream
file (fname, ios::in|ios::binary|ios::ate);
if
(file.is_open())
{ getPointer =
file.tellg();
//Get
position of the get pointer
memblock
= new
char [getPointer];
file.seekg
( 0,
ios::beg);
// repositions get pointer to beginning
getPointer
=
file.tellg();
//find new position of the get pointer
file.read
( memblock,
getPointer);
string
ID_str, refl_str;
int
numb, val , j , numbData;
double
realVal;
do
{ file.read(str2,2);
numb = Numb_Bytes(
str2[0], str2[1] ); //first read numb bytes in block
file.read(str2,2); ID_str
=
CodeID( str2[0], str2[1] ); //read block
ID
numb
=
numb -
4;
//rest of bytes to read
file.read(str2,numb);
//read rest of bytes into str2
if
( ID_str == "HEADER
Release # ")
{ val
=
Int_2u( str2[0] , str2[1]
);
//2_data_bytes=version
cout
<<" " << ID_str << val << " \n" ;
}
if
( ID_str == "BGNLIB")
{
cout
<<" " << ID_str <<
" "
;
//data_bytes=dates
}
if
( ID_str == "LIBNAME = ")
{ str2[numb]='\0'; // null terminate
str
//data_bytes=string
cout
<<" " << ID_str << str2 << " "
;
}
if
( ID_str == "FONTS = ")
{ str2[numb]='\0'; // null terminate
str
//data_bytes=string
cout
<<" " << ID_str << str2 << " \n" ;
}
if
( ID_str == "GENERATIONS")
{ val
=
Int_2u( str2[0] , str2[1]
);
//2_data_bytes=generation
cout
<<" " << ID_str <<" "<< val << " " ;
}
if
( ID_str ==
"BGNSTR")
//data_bytes=dates
{
cout
<<" " << ID_str <<
" " ;
}
if
( ID_str == "STRNAME = ")
{ str2[numb]='\0'; // null terminate
str
//data_bytes=string
cout
<<" " << ID_str << str2 << " \n" ;
}
if
( ID_str ==
"BOUNDARY")
//no data_bytes
{
cout
<<" " << ID_str << " ";
}
if
( ID_str == "LAYER = ")
{ val
=
Int_2u( str2[0] , str2[1]
);
//2_data_bytes=layer
cout
<<" " << ID_str << val << " " ;
}
if
( ID_str == "DATATYPE = ")
{ val
=
Int_2u( str2[0] , str2[1]
);
//2_data_bytes=datatype
cout
<<" " << ID_str << val << " \n" ;
}
if
( ID_str ==
"ENDEL")
//no data_bytes
{
cout
<<"" << ID_str << " \n" ;
}
if
( ID_str ==
"ENDSTR")
//no data_bytes
{
cout
<<" " << ID_str << " \n" ;
}
if
( ID_str ==
"ENDLIB")
//no data_bytes
{
cout
<<" " << ID_str << " \n" ;
}
if
( ID_str ==
"SREF")
//no data_bytes
{
cout
<<" " << ID_str <<
" " ;
}
if
( ID_str == "SNAME")
{ str2[numb]='\0'; // null terminate
str
//data_bytes=string
cout
<<" " << ID_str<<" " << str2
<< " " ;
}
if
( ID_str == "STRANS")
{ refl_str
=
"none";
val
=
Int_2u( str2[0] , str2[1]
);
//2_data_bytes=relect
if
( val != 0) refl_str = "reflect";
cout
<<" " << ID_str <<" "<< refl_str << " " ;
}
if
( ID_str == "XY = ")
{ numbData
=
numb/4;
cout
<<" " << ID_str
<<" "
;
//data_bytes(int_4) =positions
for
(j= 0; j< numbData; j=j+1)
{ val
=
Int_Sign4( str2[0+4*j], str2[1+4*j], str2[2+4*j], str2[3+4*j] );
cout
<<" " << val << "" ;
}
cout
<<"\n " ;
}
if
( ID_str == "UNITS =
")
//data_bytes(real_8) =scale
{ realVal
=
Real_8(str2[0],str2[1],str2[2],str2[3],str2[4],str2[5],str2[6],str2[7]
);
cout
<<" " << ID_str <<"" << realVal <<
" " ;
realVal
=
Real_8(str2[8],str2[9],str2[10],str2[11],str2[12],str2[13],str2[14],str2[15]
);
cout
<< realVal << " \n" ;
}
if
( ID_str == "TEXT")
{
cout
<<" " << ID_str <<
" "
; //no data_bytes
}
if
( ID_str == "TEXTTYPE")
{ val
=
Int_2u( str2[0] , str2[1]
);
//2_data_bytes=texttype
cout
<<" " << ID_str <<" " << val << " "
;
}
if
( ID_str == "PRESENTATION")
{ val
=
Int_2u( str2[0] , str2[1]
);
//2_data_bytes=presentation
cout
<<" " << ID_str <<" " << val << " "
;
}
if
( ID_str ==
"MAG")
//data_bytes(real_8) =magnitude
{ realVal
=
Real_8(str2[0],str2[1],str2[2],str2[3],str2[4],str2[5],str2[6],str2[7]
);
cout
<<" " << ID_str <<" " << realVal << "\n" ;
}
if
( ID_str ==
"ANGLE")
//data_bytes(real_8) =angle
{ realVal
=
Real_8(str2[0],str2[1],str2[2],str2[3],str2[4],str2[5],str2[6],str2[7]
);
cout
<<" " << ID_str <<" " << realVal <<
" " ;
}
if
( ID_str == "STRING")
{ str2[numb]='\0'; // null terminate
str
//data_bytes=string
cout
<<"" <<
ID_str<<" "
<< str2 << " \n " ;
}
if
( ID_str == "PATHTYPE")
{ val
=
Int_2u( str2[0] , str2[1]
);
//2_data_bytes=pathtype
cout
<<" " << ID_str <<" " << val <<
" " ;
}
if
( ID_str ==
"WIDTH")
//data_bytes(int_4) =width
{ val
=
Int_Sign4( str2[0], str2[1], str2[2], str2[3] );
cout
<<" " << ID_str <<" "
<< val << " \n" ;
}
if
( ID_str ==
"PATH")
//no data_bytes
{
cout
<<" " << ID_str <<
" " ;
}
}
while
(
!file.eof());
/* do readfile until eof */
file.close();
} else
cout
<< "Unable to open "<< fname << " \n"; //std::cout
<< "Hello, World!\n";
return 0;
}
string
CodeID(
int hi, int lo )
{
string
str = "" ;
if
( hi == 00 && lo == 02 ) str = "HEADER release # ";
if
( hi == 00 && lo == 02 ) str =
"HEADER Release # ";
if
( hi == 01 && lo == 02 ) str =
"BGNLIB"; // 0101
if
( hi == 02 && lo == 06 ) str = "LIBNAME =
"; // 0206
if
( hi == 32 && lo == 06 ) str = "FONTS =
"; // 2006
if
( hi == 34 && lo == 02 ) str =
"GENERATIONS"; // 2202
if
( hi == 03 && lo == 05 ) str = "UNITS =
"; // 0305
if
( hi == 05 && lo == 02 ) str =
"BGNSTR"; // 0502
if
( hi == 06 && lo == 06 ) str = "STRNAME =
"; // 0606
if
( hi == 8 && lo == 00 ) str =
"BOUNDARY"; // 0800
if
( hi == 13 && lo == 02 ) str = "LAYER =
"; // 0d02
if
( hi == 14 && lo == 02 ) str = "DATATYPE =
"; // 0e02
if
( hi == 16 && lo == 03 ) str = "XY =
"; // 1003
if
( hi == 17 && lo == 0 ) str =
"ENDEL"; //
1100
if
( hi == 7 && lo == 0 ) str =
"ENDSTR"; // 0700
if
( hi == 4 && lo == 0 ) str =
"ENDLIB"; // 0400
if
( hi == 10 && lo == 0 ) str =
"SREF";
// 0a00
if
( hi == 18 && lo == 6 ) str =
"SNAME"; //
1206
if
( hi == 26 && lo == 1 ) str =
"STRANS"; // 1a01
if
( hi == 12 && lo == 0 ) str =
"TEXT";
// 0c00
if
( hi == 22 && lo == 2 ) str =
"TEXTTYPE"; // 1602
if
( hi == 23 && lo == 1 ) str =
"PRESENTATION"; // 1701
if
( hi == 27 && lo == 5 ) str =
"MAG";
// 1b05
if
( hi == 25 && lo == 6 ) str =
"STRING"; // 1906
if
( hi == 33 && lo == 2 ) str =
"PATHTYPE"; // 2102
if
( hi == 15 && lo == 3 ) str =
"WIDTH"; //
0f03
if
( hi == 9 && lo == 0 ) str =
"PATH";
// 0900
if
( hi == 28 && lo == 5 ) str =
"ANGLE"; //
1C05
return str;
}
double
Real_8(int
X0, int X1, int X2, int X3,int X4, int X5, int X6, int X7 )
{
if
( X0 <0 ) X0 = X0+256;
if
( X1 <0 ) X1 = X1+256;
if
( X2 <0 ) X2 = X2+256;
if
( X3 <0 ) X3 = X3+256;
if
( X4 <0 ) X4 = X4+256;
if
( X5 <0 ) X5 = X5+256;
if
( X6 <0 ) X6 = X6+256;
if
( X7 <0 ) X7 = X7+256;
int
exp , i ,bit,testbit, scalebit;
exp
= (X0 &
127);
// will bit mask to = 01111111
exp
=
0;
for
(i=1; i< 8 ; i++)
{ bit
=
(int) pow(2,7-i);
exp
=
exp + (X0 & bit);
}
exp
=
exp-65;
// add offset
double
matval,matvalb , value;
matval
= 0;
for
(i=0; i< 8 ; i++)
{ bit
=
pow(2,7-i);
scalebit
=
pow(2,i);
testbit
= (X1 &
bit);
matvalb
= (double)
8*(X1 & bit)/(bit*scalebit);
matval
= matval +
matvalb;
matvalb
= (double)
8*(X2 & bit)/(256*bit*scalebit);
matval
= matval +
matvalb;
matvalb
= (double)
8*(X3 & bit)/(256*256*bit*scalebit);
matval
= matval +
matvalb;
} value
=
matval*pow(16,exp);
return value;
}
int
Int_Sign4(
int first, int second, int third, int forth )
{
int
numb ;
if
( second <0 ) second = second+256;
if
( third <0 ) third = third +256;
if
( forth <0 ) forth = forth +256;
numb
=
256*(256*(256*first + second)+third )+forth;
return numb;
}
int
Numb_Bytes(
int hi, int lo )
{
int
numb ;
if
( hi <0 ) hi = hi+256;
if
( lo <0 ) lo = lo+256;
numb =256*hi + lo;
return numb;
}
unsigned
Int_2u(
unsigned hi, unsigned lo )
{
int
numb ;
numb =256*hi + lo;
//
cout
<<hi<<" "<<lo<<""<<numb<< "
\n" ;
return numb;
}
============Compile/RUN========================
[Session started at
2009-05-07 16:10:43 -0700.]
M1TEST.SF is the file being read
HEADER Release #
3
BGNLIB LIBNAME =
M1TEST.DB FONTS = GDSII:CALMAFONT.TX
GENERATIONS 3 UNITS = 0.001 1e-09
BGNSTR STRNAME =
M1TEST
BOUNDARY LAYER =
8 DATATYPE = 0
XY =
-23100 32500 -23100 12500 -6400 12500 -6400 32500 -23100 32500
ENDEL
PATH
LAYER = 8 DATATYPE = 0
PATHTYPE 2 WIDTH
1400
XY =
-12100 23000 25600 23000 25600 11700 40400 11700
ENDEL
TEXT
LAYER = 8 TEXTTYPE 0 PRESENTATION
8 STRANS none MAG 3
XY =
-17000 25400
STRING VCC
ENDEL
TEXT
LAYER = 8 TEXTTYPE 0 PRESENTATION
8 STRANS none MAG 2
ANGLE 90 XY
= -18700
14400
STRING VCC2
ENDEL
SREF
SNAME AT_ZERO STRANS none XY
= 0 0
ENDEL
PATH
LAYER = 10 DATATYPE = 0
PATHTYPE 2 WIDTH
1800
XY =
-700 5900 6200 5900 6200 -5300
ENDEL
ENDSTR
BGNSTR STRNAME =
AT_ZERO
BOUNDARY LAYER =
50 DATATYPE = 1
XY =
-1000 0 -1000 -1000 0 -1000 0 0 -1000 0
ENDEL
BOUNDARY LAYER =
50 DATATYPE = 1
XY =
0 1000 0 0 1000 0 1000 1000 0 1000
ENDEL
ENDSTR
ENDLIB
============Convert_BMP========
#include
<iostream>
#include
<fstream>
#include
<math.h>
using namespace std;
ifstream::pos_type getPointer;
const
char
*fname;
char
str[60];
int
Int_Sign4( int first, int second, int third, int forth ) ;
int
Int_USign4( int first, int second, int third, int forth );
int
Int_USign2( int first, int second );
int
Int_Sign2( int first, int second );
int
Int_USign1( int first);
int
Int_USign1s( int first);
double
Extend_10(int X0,int X1,int X2,int X3,int X4,int X5,int X6,int X7,int
X8,int X9 );
int
main (int argc, char * const argv[])
{
int
val , i , j ;
fname
=
"000.bmp";
cout
<<" "<< fname << " " ;
ifstream
file (fname, ios::in|ios::binary|ios::ate);
if
(file.is_open())
{ getPointer =
file.tellg(); cout << "
file size is " << getPointer << " \n";
file.seekg
( 0,
ios::beg); //
repositions get pointer to beginning
}
cout <<
"ADDR
TYPE
VALUE \n";
getPointer
=
file.tellg(); cout << getPointer <<
" BMP_HEADER ";
file.read(str,2); str[2]= '\0';
cout <<" " << str << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" FILE_SIZE ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0] );
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" ALWAYS_ZERO ";
file.read(str,2);
val
=
Int_USign2( str[1], str[0] );
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" ALWAYS_ZERO ";
file.read(str,2);
val
=
Int_USign2( str[1], str[0] );
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" OFFSET2DATA ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" BM_HEADSIZE ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" WIDTH_PIXEL ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" HEIGHT_PIXL ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" NUMB_PLANES ";
file.read(str,2);
val
=
Int_USign2( str[1], str[0] );
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" BITS_PIXEL ";
file.read(str,2);
val
=
Int_USign2( str[1], str[0] );
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" COMPRESS ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" IMAGESIZE ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" PIXEL/METER ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" PIXEL/METER ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" NUMB_COLORS ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" IMPORTANTC ";
file.read(str,4);
val
=
Int_USign4( str[3], str[2], str[1], str[0]);
cout <<" " << val << " \n" ;
getPointer
=
file.tellg(); cout << getPointer <<
" BEGIN_DATA \n";
cout
<<
" bgr bgr bgr bgr bgr
bgr bgr bgr bgr bgr pad\n" ;
for
(j=0; j< 10 ; j++)
{ cout
<<
"row "<< j <<" " ;
for
(i=0; i< 10 ; i++)
{ file.read(str,1); val = Int_USign1s( str[0]);cout <<""
<< val << "" ;
file.read(str,1); val = Int_USign1s( str[0]);cout
<<"" << val << "" ;
file.read(str,1); val = Int_USign1s( str[0]);cout
<<"" << val << " " ;
}
for
(i=0; i< 2 ; i++)
{ file.read(str,1); val = Int_USign1s( str[0]);cout <<""
<< val << " " ;
} cout <<" \n" ;
}
return 0;
}
double
Extend_10(int
X0,int X1,int X2,int X3,int X4,int X5,int X6,int X7,int
X8,int X9 )
{
if
( X0 <0 ) X0 = X0+256;
if
( X1 <0 ) X1 = X1+256;
if
( X2 <0 ) X2 = X2+256;
if
( X3 <0 ) X3 = X3+256;
if
( X4 <0 ) X4 = X4+256;
if
( X5 <0 ) X5 = X5+256;
if
( X6 <0 ) X6 = X6+256;
if
( X7 <0 ) X7 = X7+256;
if
( X8 <0 ) X8 = X8+256;
if
( X9 <0 ) X9 = X9+256;
int
exp , i ,bit,testbit, scalebit;
exp
= (X0 & 127); //
mask = 01111111
exp
=
0;
//cout << " exp "<< exp <<"
<< \n" ;
for
(i=1; i< 8 ; i++)
{ bit
=
(int) pow(2,15-i);
exp
=
exp + (X0 & bit);
}
for
(i=0; i< 8 ; i++)
{ bit
=
(int) pow(2,7-i);
exp
=
exp + (X1 & bit);
}
exp
= exp +1; // add offset
double
matval,matvalb , value;
matval
= 0;
for
(i=0; i< 8 ; i++)
{ bit
=
pow(2,7-i);
scalebit
=
pow(2,i+3);
testbit
= (X2 &
bit);
matvalb
= (double)
8*(X2 & bit)/(bit*scalebit);
matval
= matval +
matvalb;
matvalb
= (double)
8*(X3 & bit)/(256*bit*scalebit);
matval
= matval +
matvalb;
matvalb
= (double)
8*(X4 & bit)/(256*256*bit*scalebit);
matval
= matval +
matvalb;
} value
=
matval*pow(2,exp);
return value;
}
int
Int_Sign2(
int first, int second )
{
int
numb ;
if
( second <0 ) second = second+256;
numb
=
256*first + second;
return numb;
}
int
Int_USign2(
int first, int second )
{
int
numb ;
if
( first <0 ) first = first+256;
if
( second <0 ) second = second+256;
numb
=
256*first + second;
return numb;
}
int
Int_USign1(
int first)
{
int
numb ;
if
( first <0 ) first = first+256;
numb
=
first ;
return numb;
}
int
Int_USign1s(
int first)
{
int
numb ;
if
( first <0 ) first = first+256;
numb
=
first/255 ;
return numb;
}
int
Int_USign4(
int first, int second, int third, int forth )
{
int
numb ;
if
( first <0 ) first = first +256;
if
( second <0 ) second = second+256;
if
( third <0 ) third = third +256;
if
( forth <0 ) forth = forth +256;
numb
=
256*(256*(256*first + second)+third )+forth;
return numb;
}
int
Int_Sign4(
int first, int second, int third, int forth )
{
int
numb ;
if
( second <0 ) second = second+256;
if
( third <0 ) third = third +256;
if
( forth <0 ) forth = forth +256;
numb
=
256*(256*(256*first + second)+third )+forth;
// cout << " " << first << " " <<
second<< " " << third<< " " << forth << "
\n";
return numb;
}
=================Compile/RUN========================
[Session started at 2009-01-03 15:53:45 -0800.]
000.bmp file size
is 374
ADDR TYPE
VALUE
0 BMP_HEADER BM
2 FILE_SIZE 374
6 ALWAYS_ZERO 0
8 ALWAYS_ZERO 0
10 OFFSET2DATA 54
14 BM_HEADSIZE 40
18 WIDTH_PIXEL 10
22 HEIGHT_PIXL 10
26 NUMB_PLANES 1
28 BITS_PIXEL 24
30 COMPRESS 0
34 IMAGESIZE 320
38 PIXEL/METER 2835
42 PIXEL/METER 2835
46 NUMB_COLORS 0
50 IMPORTANTC 0
54 BEGIN_DATA
bgr bgr bgr bgr bgr bgr bgr bgr bgr bgr
pad
row 0 111 111 111 111 111 111 111 111 111 111 0 0
row 1 111 111 111 111 111 111 111 111 111 111 0 0
row 2 111 111 111 111 111 111 111 111 111 111 0 0
row 3 111 111 111 111 111 111 111 111 111 111 0 0
row 4 111 000 111 001 111 010 111 100 111 011 0 0
row 5 111 000 111 001 111 010 111 100 111 011 0 0
row 6 111 000 111 001 111 010 111 100 111 011 0 0
row 7 111 000 111 001 111 010 111 100 111 011 0 0
row 8 111 000 111 001 111 010 111 100 111 011 0 0
row 9 111 111 111 111 111 111 111 111 111 111 0 0
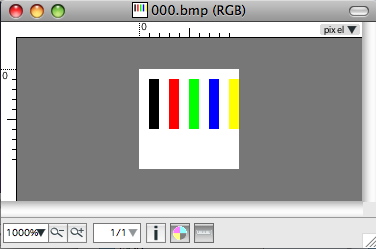
//========reading a complete binary
file========
#include
<iostream>
#include
<fstream>
using
namespace std;
ifstream::pos_type size;
char
*
memblock;
int
main ()
{
int
i ,j , h, l , z , m, s;
ifstream file
("/Users/donsauer/Desktop/cmdtests/3N45E007.hgt",
ios::in|ios::binary|ios::ate);
ofstream myfile
("/Users/donsauer/Desktop/1.txt");
if
(file.is_open())
{ size
=
file.tellg();
cout
<< " file size is " << size ;
memblock
= new char [size];
file.seekg (0, ios::beg);
file.read (memblock, size);
file.close();
cout
<< " the complete file content is in memory \n" ;
s=
3;
m
=
1201*s ; // 7200 points per row file 25934402
is 3602X7200
m
=
3601;
for
(j=0; j< 180*2; j=j+4)
{
for
(i=2250; i< 2460; i=i+2)
{ h
=
(int) memblock[ 2*i +m*j];
if
( h < 0 ) h=h+256;
l
=
(int) memblock[2*i+1 +m*j];
if
( l < 0 ) l=l+256;
z
=
(256*h +l)-2000;
myfile
<< z << " " ;
}
myfile
<< "\n" ;
}
myfile.close()
delete[] memblock;
} else
cout << "Unable
to open file";
return
0;
}
//========reading a complete text
file========
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main () {
string line;
ifstream myfile
("/Users/donsauer/Desktop/zzzz/cmdtests/example.txt");
if (myfile.is_open())
{
while (! myfile.eof() )
{
getline (myfile,line);
cout << line << endl;
}
myfile.close();
}
else cout << "Unable to
open file";
return 0;
}
//========write a complete text
file========
#include
<iostream>
#include
<fstream>
using
namespace std;
int
main (int argc, char * const argv[])
{ ofstream myfile
("/Users/donsauer/Desktop/cmdtests/example.txt");
if
(myfile.is_open())
{ myfile
<< "This is a line.\n";
myfile
<< "This is another line.\n";
myfile.close();
} else cout << "Unable
to open file";
return 0;
std::cout <<
"Hello, World!\n"; // insert code here...
return 0;
}
//========obtain file size========
// obtaining file size
#include
<iostream>
#include
<fstream>
using
namespace std;
int
main ()
{
long
begin,end;
ifstream
myfile ("/Users/donsauer/Desktop/cmdtests/example.txt");
begin
=
myfile.tellg();
myfile.seekg (0, ios::end);
end
=
myfile.tellg();
myfile.close();
cout
<< "size is: " << (end-begin) << " bytes.\n";
return
0;
}